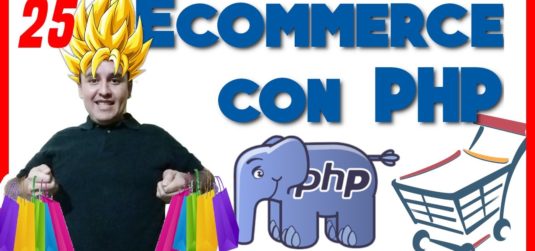
25.-Crear área de datos de envió de nuestro ecommerce en PHP
En este tutorial vamos crear área de datos de envió de nuestro ecommerce en PHP 🛒 en donde vamos a poner los datos de la persona que va a recibir los productos que vamos a comprar.
Codigo: https://github.com/programadornovato/ecommerce/commit/8db5f41a1e8d4603c1b2748fd0a34a66590dff65
admin/js/ecommerce.js
$(document).ready(function () { $.ajax({ type: "post", url: "ajax/leerCarrito.php", dataType: "json", success: function (response) { llenaCarrito(response); } }); $.ajax({ type: "post", url: "ajax/leerCarrito.php", dataType: "json", success: function (response) { llenarTablaCarrito(response); } }); function llenarTablaCarrito(response){ $("#tablaCarrito tbody").text(""); var TOTAL=0; response.forEach(element => { var precio=parseFloat(element['precio']); var totalProd=element['cantidad']*precio; TOTAL=TOTAL+totalProd; $("#tablaCarrito tbody").append( ` <tr> <td><img src="${element['web_path']}" class="img-size-50"/></td> <td>${element['nombre']}</td> <td> ${element['cantidad']} <button type="button" class="btn-xs btn-primary mas" data-id="${element['id']}" data-tipo="mas" >+</button> <button type="button" class="btn-xs btn-danger menos" data-id="${element['id']}" data-tipo="menos" >-</button> </td> <td>$${precio.toFixed(2)}</td> <td>$${totalProd.toFixed(2)}</td> <td><i class="fa fa-trash text-danger borrarProducto" data-id="${element['id']}" ></i></td> <tr> ` ); }); $("#tablaCarrito tbody").append( ` <tr> <td colspan="4" class="text-right"><strong>Total:</strong></td> <td>$${TOTAL.toFixed(2)}</td> <td></td> <tr> ` ); } $(document).on("click",".mas,.menos",function(e){ e.preventDefault(); var id=$(this).data('id'); var tipo=$(this).data('tipo'); $.ajax({ type: "post", url: "ajax/cambiaCantidadProductos.php", data: {"id":id,"tipo":tipo}, dataType: "json", success: function (response) { llenarTablaCarrito(response); llenaCarrito(response); } }); }); $(document).on("click",".borrarProducto",function(e){ e.preventDefault(); var id=$(this).data('id'); $.ajax({ type: "post", url: "ajax/borrarProductoCarrito.php", data: {"id":id}, dataType: "json", success: function (response) { llenarTablaCarrito(response); llenaCarrito(response); } }); }); $("#agregarCarrito").click(function (e) { e.preventDefault(); var id=$(this).data('id'); var nombre=$(this).data('nombre'); var web_path=$(this).data('web_path'); var cantidad=$("#cantidadProducto").val(); var precio=$(this).data('precio'); $.ajax({ type: "post", url: "ajax/agregarCarrito.php", data: {"id":id,"nombre":nombre,"web_path":web_path,"cantidad":cantidad,"precio":precio}, dataType: "json", success: function (response) { llenaCarrito(response); $("#badgeProducto").hide(500).show(500).hide(500).show(500).hide(500).show(500); $("#iconoCarrito").click(); } }); }); function llenaCarrito(response){ var cantidad=Object.keys(response).length; if(cantidad>0){ $("#badgeProducto").text(cantidad); }else{ $("#badgeProducto").text(""); } $("#listaCarrito").text(""); response.forEach(element => { $("#listaCarrito").append( ` <a href="index.php?modulo=detalleproducto&id=${element['id']}" class="dropdown-item"> <!-- Message Start --> <div class="media"> <img src="${element['web_path']}" class="img-size-50 mr-3 img-circle"> <div class="media-body"> <h3 class="dropdown-item-title"> ${element['nombre']} <span class="float-right text-sm text-primary"><i class="fas fa-eye"></i></span> </h3> <p class="text-sm">Cantidad ${element['cantidad']}</p> </div> </div> <!-- Message End --> </a> <div class="dropdown-divider"></div> ` ); }); $("#listaCarrito").append( ` <a href="index.php?modulo=carrito" class="dropdown-item dropdown-footer text-primary"> Ver carrito <i class="fa fa-cart-plus"></i> </a> <div class="dropdown-divider"></div> <a href="#" class="dropdown-item dropdown-footer text-danger" id="borrarCarrito"> Borrar carrito <i class="fa fa-trash"></i> </a> ` ); } $(document).on("click","#borrarCarrito",function(e){ e.preventDefault(); $.ajax({ type: "post", url: "ajax/borrarCarrito.php", dataType: "json", success: function (response) { llenaCarrito(response); } }); }); var nombreRec=$("#nombreRec").val(); var emailRec=$("#emailRec").val(); var direccionRec=$("#direccionRec").val(); $("#jalar").click(function (e) { var nombreCli=$("#nombreCli").val(); var emailCli=$("#emailCli").val(); var direccionCli=$("#direccionCli").val(); if( $(this).prop("checked")==true ){ $("#nombreRec").val(nombreCli); $("#emailRec").val(emailCli); $("#direccionRec").val(direccionCli); }else{ $("#nombreRec").val(nombreRec); $("#emailRec").val(emailRec); $("#direccionRec").val(direccionRec); } }); });
carrito.php
<table class="table table-striped table-inverse" id="tablaCarrito" > <thead class="thead-inverse"> <tr> <th>Imagen</th> <th>Nombre</th> <th>Cantidad</th> <th>Precio</th> <th>Total</th> <th></th> </tr> </thead> <tbody> </tbody> </table> <a class="btn btn-warning" href="index.php?modulo=productos" role="button">Ir a productos</a> <a class="btn btn-primary float-right" href="index.php?modulo=envio" role="button">Ir a datos de envio</a>
envio.php
<?php if (isset($_SESSION['idCliente'])) { ?> <div class="container mt-3"> <div class="row"> <div class="col-6"> <h3>Datos del cliente</h3> <div class="form-group"> <label for="">Nombre</label> <input type="text" name="nombreCli" id="nombreCli" class="form-control"> </div> <div class="form-group"> <label for="">Email</label> <input type="email" name="emailCli" id="emailCli" class="form-control"> </div> <div class="form-group"> <label for="">Direccion</label> <textarea name="direccionCli" id="direccionCli" class="form-control" row="3"></textarea> </div> </div> <div class="col-6"> <h3>Datos de quien recibe</h3> <div class="form-group"> <label for="">Nombre</label> <input type="text" name="nombreRec" id="nombreRec" class="form-control"> </div> <div class="form-group"> <label for="">Email</label> <input type="email" name="emailRec" id="emailRec" class="form-control"> </div> <div class="form-group"> <label for="">Direccion</label> <textarea name="direccionRec" id="direccionRec" class="form-control" row="3"></textarea> </div> <div class="form-check"> <label class="form-check-label"> <input type="checkbox" class="form-check-input" id="jalar" > Jalar datos del cliente </label> </div> </div> </div> </div> <?php } else { ?> <div class="mt-5 text-center"> Debe <a href="login.php">loguearse</a> o <a href="registro.php">registrarse</a> </div> <?php } ?>
index.php
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <title>My ecommerce by pn</title> <!-- Tell the browser to be responsive to screen width --> <meta name="viewport" content="width=device-width, initial-scale=1"> <!-- Font Awesome --> <link rel="stylesheet" href="admin/plugins/fontawesome-free/css/all.min.css"> <!-- Ionicons --> <link rel="stylesheet" href="https://code.ionicframework.com/ionicons/2.0.1/css/ionicons.min.css"> <!-- Theme style --> <link rel="stylesheet" href="admin/dist/css/adminlte.min.css"> <!-- Daterange picker --> <link rel="stylesheet" href="admin/plugins/daterangepicker/daterangepicker.css"> <!-- Google Font: Source Sans Pro --> <link href="https://fonts.googleapis.com/css?family=Source+Sans+Pro:300,400,400i,700" rel="stylesheet"> <?php session_start(); $accion=$_REQUEST['accion']??''; if($accion=='cerrar'){ session_destroy(); header("Refresh:0"); } ?> </head> <body> <?php include_once "admin/db_ecommerce.php"; $con = mysqli_connect($host, $user, $pass, $db); ?> <div class="container"> <div class="row"> <div class="col-12"> <?php include_once "menu.php"; $modulo=$_REQUEST['modulo']??''; if($modulo=="productos" || $modulo=="" ){ include_once "productos.php"; } if( $modulo=="detalleproducto" ){ include_once "detalleProducto.php"; } if( $modulo=="carrito" ){ include_once "carrito.php"; } if( $modulo=="envio" ){ include_once "envio.php"; } ?> </div> </div> </div> <!-- jQuery --> <script src="admin/plugins/jquery/jquery.min.js"></script> <!-- jQuery UI 1.11.4 --> <script src="admin/plugins/jquery-ui/jquery-ui.min.js"></script> <!-- Bootstrap 4 --> <script src="admin/plugins/bootstrap/js/bootstrap.bundle.min.js"></script> <!-- daterangepicker --> <script src="admin/plugins/moment/moment.min.js"></script> <script src="admin/plugins/daterangepicker/daterangepicker.js"></script> <!-- AdminLTE App --> <script src="admin/dist/js/adminlte.js"></script> <!-- AdminLTE dashboard demo (This is only for demo purposes) --> <script src="admin/dist/js/pages/dashboard.js"></script> <script src="admin/js/ecommerce.js"></script> </body> </html>
🎦Curso de PHP🐘 y MySql🐬: https://www.youtube.com/playlist?list=PLCTD_CpMeEKS2Dvb-WNrAuDAXObB8GzJ0
🎦[Curso] Laravel Tutorial en Español: https://www.youtube.com/playlist?list=PLCTD_CpMeEKQcVcM4u4qddLYRE37S_0XS
🎦Curso]Ajax con Jquery de 0 a 100 🌇: https://www.youtube.com/watch?v=52yI0xiB73A&list=PLCTD_CpMeEKSYJ1J15M8PknOMwOpeqsXz
🎦Mysql configurar una replicación maestro – esclavo 🐬: https://www.youtube.com/watch?v=RY-EdBOJWEs
🎦[Curso] Visual Studio Code 🆚 de 0 a 100: https://www.youtube.com/playlist?list=PLCTD_CpMeEKQbdlT8efsS-veXuvYZ1UWn
🎦[Curso] Bootstrap de 0 a 100 🌈: https://www.youtube.com/playlist?list=PLCTD_CpMeEKSVmsZJIumVvfDviuW-c9AT
🎦[Curso] HTML y CSS de 0 a 100 🌐: https://www.youtube.com/playlist?list=PLCTD_CpMeEKS1SmufBGPOV1mjNfCiLwek
🎦 Esta lista de reproducción: https://www.youtube.com/playlist?list=PLCTD_CpMeEKQhRiJx7Wv3pM3rYvT9_CS9 .
Codigos en gdrive: https://drive.google.com/file/d/1QW8ExkL8eS7nQ5HTDvUuSkkGJMSmecGV/view?usp=sharing
Gracias por apoyar este canal: https://www.patreon.com/programadornovato?fan_landing=true
🔗 Facebook: https://facebook.com/ProgramadorNovatoOficial
🔗 Twitter: https://twitter.com/programadornova
🔗 Linkedin: https://www.linkedin.com/in/programadornovato/
🔗 Instagram: https://www.instagram.com/programadornovato/
<<Anterior tutorial Siguiente tutorial >>
Ave que vuela, a la cazuela.