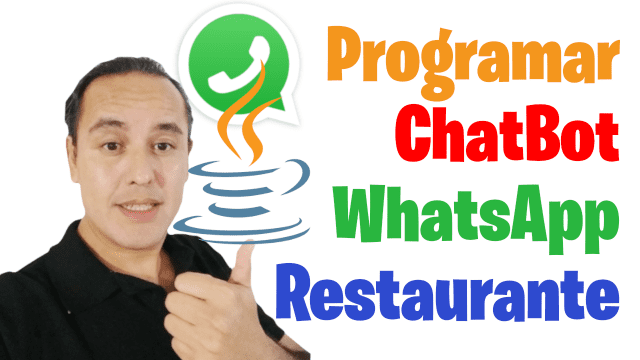
Programar en Java un ChatBot WhatsApp para restaurante
En el tutorial anterior aprendimos a instalar nuestro ChatBot WhatsApp para restaurante, ahora en este tutorial aprenderemos a Programar en Java un ChatBot WhatsApp para restaurante, a estas alturas ya debemos tener Java JDK (en mi caso es la versión 11) y NetBeans (en mi caso la versión 12) o Eclipse si seguiremos estos pasos:
Configuramos el proyecto
Click en nuevo proyecto y seleccionamos Java with Maven y click en Next
/
Le colocamos un nombre en mi caso ChatBotRestaurante y Finish
Abrimos el archivo pom.xml y pegamos estas dependencias:
<dependencies> <dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium-api</artifactId> <version>4.1.0</version> <type>jar</type> </dependency> <dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium-chrome-driver</artifactId> <version>4.1.0</version> <type>jar</type> </dependency> <dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium-support</artifactId> <version>4.1.0</version> <type>jar</type> </dependency> <dependency> <groupId>backport-util-concurrent</groupId> <artifactId>backport-util-concurrent</artifactId> <version>3.1</version> <type>jar</type> </dependency> <dependency> <groupId>com.opencsv</groupId> <artifactId>opencsv</artifactId> <version>3.7</version> </dependency> <dependency> <groupId>io.github.bonigarcia</groupId> <artifactId>webdrivermanager</artifactId> <version>5.3.1</version> <type>jar</type> </dependency> </dependencies>
Creamos el proyecto
Click derecho en el paquete del proyecto -> New -> JFrame
Colocamos cualquier nombre en mi caso ChatBotRestaurante y click en Finish
Agregamos un boton llamado btnAbrirWA y un checkBox llamado jCheckBox1
Insertamos codigo
Creamos la carpeta chromeWA y colocamos los archivos datos.csv y menu.csv
Agregaos un evento click en btnAbrirWA y en jCheckBox1 y colocamos este código
private void btnAbrirWAActionPerformed(java.awt.event.ActionEvent evt) { abrirNavegador(); } private void jCheckBox1ActionPerformed(java.awt.event.ActionEvent evt) { activarWA(); }
Importamos estas dependencias:
import com.google.common.collect.Streams; import java.io.BufferedReader; import java.io.File; import java.io.FileReader; import java.io.IOException; import java.text.SimpleDateFormat; import java.time.LocalDateTime; import java.time.temporal.ChronoUnit; import java.util.ArrayList; import java.util.Arrays; import java.util.List; import java.util.Locale; import java.util.concurrent.TimeUnit; import java.util.logging.Level; import java.util.logging.Logger; import javax.swing.JOptionPane; import org.openqa.selenium.By; import org.openqa.selenium.JavascriptExecutor; import org.openqa.selenium.Keys; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.WindowType; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import org.openqa.selenium.support.ui.Select; import org.openqa.selenium.support.ui.WebDriverWait; import com.opencsv.CSVReader; import com.opencsv.CSVWriter; import java.time.DayOfWeek; import java.time.LocalDate; import java.util.NoSuchElementException; import io.github.bonigarcia.wdm.WebDriverManager;
Y creamos estas variables y funciones:
//DECLARAMOS EL WEBDRIVER QUE CONTROLARA EL CHROME WebDriver driver; //XPATH DEL AREA DE ESCRITURA DE WA String inputTextEnviarWA = "//*[@id=\"main\"]/footer/div[1]/div/span[2]/div/div[2]/div[1]/div/div[1]/p"; ArrayList<String> tabs; //NUMERO DE CONTACTOS QUE ESTAREMOS MANEJANDO int cantTabs = 0; //DIRECCION ACTUAL DEL PROGRAMA String localPath = ""; //*********MULTIUSUARIO******************* //LISTA DE CONTACTOS QUE MANEJAREMOS String[] contactos = new String[100]; int[] etapa = new int[100]; //USUARIO SELECCIONADO O TAB SELECCIONADA int usuarioSel = 0; //MENSAJES RECIBIDOS DESDE WA String[] mensajesRecibidos = new String[100]; /* VARIABLES DE EXCEL */ String saludo; String telefono; String direccion; String domicilio; String pagina; String lunes; String martes; String miercoles; String jueves; String viernes; String sabado; String domingo; //CREAMOS UN ARREGLO QUE ALAMACENARA LOS DATOS DEL MENU List<String[]> registrosMenu = new ArrayList<>(); public ChatBotRestaurante() { //OBTENEMOS LA DIRECCION ACTUAL DEL PROGRAMA try { localPath = new File(".").getCanonicalPath(); } catch (IOException ex) { Logger.getLogger(ChatBotRestaurante.class.getName()).log(Level.SEVERE, null, ex); } initComponents(); leemosMenu(); leemosDatos(); } public void abrirNavegador() { try { /* String SO = System.getProperty("os.name"); //SI EL SISTEMA OPERATIVO ES WINDOWS if (SO.equals("Windows 10")) { //LEEMOS EL CHROMEDRIVER QUE ESTA EN LA MISMA DIRECCION DEL PROGRAMA System.setProperty("webdriver.chrome.driver", localPath + "\\chromedriver.exe"); } else { System.setProperty("webdriver.chrome.driver", localPath + "/chromedriver"); } */ WebDriverManager.chromedriver().setup(); //INICIALIZAMOS LS OPCIONES DE CHROME ChromeOptions optionsGoo = new ChromeOptions(); //PERMITIMOS LA PROPIEDAD NO-SANDBOX PARA EVITAR PROBLEMAS EN LINUX optionsGoo.addArguments("--no-sandbox"); //DESHABILITAMOS LAS NOTIFICACIONES optionsGoo.addArguments("--disable-notifications"); optionsGoo.addArguments("Zoom 70%"); //GUARDAMOS LA SESION EN LA CARPETA CHROMEWA optionsGoo.addArguments("--user-data-dir=" + localPath + "\\chromeWA"); //INSTANCIAMOS UN NUEVO CHROMEDRIVER driver = new ChromeDriver(optionsGoo); driver.manage().timeouts().implicitlyWait(5, TimeUnit.SECONDS); //ABRIMOS WHATSAPP driver.get("https://web.whatsapp.com"); } catch (NoSuchElementException e) { Object[] options = {"Continuar", "Detener"}; int res = JOptionPane.showOptionDialog(null, "Desea continuar o detener? \n error= " + e, "Warning", JOptionPane.DEFAULT_OPTION, JOptionPane.WARNING_MESSAGE, null, options, options[0]); if (res == 1) { driver.quit(); } } catch (Exception e) { Object[] options = {"Continuar", "Detener"}; int res = JOptionPane.showOptionDialog(null, "Version de chrome no compatible porfavor descargue la version de chrome que corresponde \n error= " + e, "Warning", JOptionPane.DEFAULT_OPTION, JOptionPane.WARNING_MESSAGE, null, options, options[0]); if (res == 1) { driver.quit(); } } } public void activarWA() { //HORA EN LA QUE INICIO EL USUARIO while (jCheckBox1.isSelected() == true) { //REVISAMOS QUE EXISTAN NUEVO MENSAJES EN EL CHAT DE WA revisaWA(); pausa(1000); } } public void revisaWA() { //VARIABLE QUE ALMACENA LA TAB DE CHROME QUE NOS POSICIONAREMOS int tabSeleccionada = 0; //SI HAY UN MENSAJE EN WA if (driver.findElements(By.className("aumms1qt")).size() > 0) { //BUSCAMOS EL NOMBRE DEL CONTACTO String nombreContacto = driver.findElement(By.className("aumms1qt")).findElement(By.xpath("./../../../../..")).findElement(By.className("zoWT4")).getText(); //OBTENEMOS LA CANTIDAD MENSAJES ENVIADOS int cantNuevosMensajes = Integer.parseInt(driver.findElement(By.className("aumms1qt")).getText()); //DAMOS CLICK EN EL CONTACTO PARA ABRIR EL CHAT driver.findElement(By.className("aumms1qt")).findElement(By.xpath("./../../../../..")).findElement(By.className("zoWT4")).click(); //OBTENEMOS TODOS LOS MENSAJES List<WebElement> webElementsMsgWA = driver.findElements(By.className("_22Msk")); //OBTENEMOS TODAS LAS TABS tabs = new ArrayList<String>(driver.getWindowHandles()); boolean contactoEncontrado = false; //VALIDAMOS QUE EL NOMBRE DE CONTACTO NO ESTE EN LA LISTA DE CONTACTOS DE LO CONTRARIO NOS POSICIONAMOS EN EL ID DEL CONTACTO for (int tabSel = 1; tabSel <= cantTabs; tabSel++) { //SI EL CONTACTO YA EXISTE EN LA LISTA if (nombreContacto.equals(contactos[tabSel])) { //ACTIVAMOS LA BANDERA Y DECMOS QUE SI ENCONTRAMOS EL CONTACTO contactoEncontrado = true; usuarioSel = tabSel; //ROMPEMOS EL CICLO break; } } //SI NO SE ENCONTRO EL CONTACTO LO CREAMOS if (contactoEncontrado == false) { //AUMENTAMOS EL NUMERO DE CONTACTOS cantTabs++; //ASIGNAMOS EL NOMBRE DEL CONTACTO A LA LISTA DE CONTACTOS contactos[cantTabs] = nombreContacto; //OBTENEMOS EL ID DEL USUARIO SELECCIONADO usuarioSel = cantTabs; //ASIGANOS LA ETAPA DEL USUARIO QUE ES LA 1 (ENVIAR TEXTOS D ELOS SORTEOS) etapa[usuarioSel] = 1; } //VARIABLE QUE ALMACENARA LOS ULTIMOS MENSAJE ENVIADOS mensajesRecibidos[usuarioSel] = ""; //CICLO QUE UNIRA TODOS LOS ULTIMOS MENSAJES for (int i = cantNuevosMensajes; i >= 1; i--) { //OBTENEMOS CADA MENSAJE String ultimoMensaje = webElementsMsgWA.get(webElementsMsgWA.size() - i).findElement(By.className("i0jNr")).getText(); //UNIMOS LOS MENSAJES mensajesRecibidos[usuarioSel] = mensajesRecibidos[usuarioSel] + " " + ultimoMensaje; } try { if (etapa[usuarioSel] == 1) { //ETAPA 1 DONDE SE ENVIAN LOS MENSAJES DE LOS DIFERENTES SORTEOS etapa1(); return; } else if (etapa[usuarioSel] == 2) { //VALIDAMOS QUE SI SEA UN NUMERO DEL SORTEO Y PREGUNTAMOS SI QUIERE UN NUMERO AL AZAR Y QUIERE SELECCIONAR SU NUMERO //SI NO LLAMAMOS LA ETAPA 1 PARA QUE ENVIE POR WA LOS SORTEOS etapa2(); return; } if (etapa[usuarioSel] == 3) { //VALIDAMOS QUE SI SEA UN NUMERO DEL SORTEO Y PREGUNTAMOS SI QUIERE UN NUMERO AL AZAR Y QUIERE SELECCIONAR SU NUMERO //SI NO LLAMAMOS LA ETAPA 2 PARA QUE ENVIE POR WA LOS SORTEOS etapa3(); return; } } catch (Exception e) { java.util.logging.Logger.getLogger(ChatBotRestaurante.class.getName()).log(java.util.logging.Level.SEVERE, null, e); } } else { } } //Etapa 1 Donde se envian los mensajes de los diferentes sorteos public void etapa1() { //ABRIMOS EL TAB DEL CONTACTO String listaOpciones = saludo + "\n1.-Menu del dia de hoy\n2.-Menu de otro dia\n3.-Horarios\n4.-Acerca de nosotros\n"; //ESCRIBIMOS EL MENSAJE driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys(listaOpciones); pausa(500); //CERRAMOS CHAT DE WA driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys(Keys.ESCAPE); //PASAMOS A LA SIGUIENTE ETAPA etapa[usuarioSel] = 2; } public void etapa2() { //VARIABLE QUE RECIBE LAS OPCIONES COLOCADAS POR EL USUARIO DE WA int opcion; //QUITAMOS ESPACIOS EN BLANCO String mensajesRecibidosSinEspacios = mensajesRecibidos[usuarioSel].replace(" ", ""); //String mensajesRecibidosSinEspacios = "2"; //VALIDAMO SI REALMENTE SE ENVIO UN NUMERO if (isNumeric(mensajesRecibidosSinEspacios)) { opcion = Integer.parseInt(mensajesRecibidosSinEspacios); //SI LA OPCION ESTA ENTRE 1 Y 4 if (opcion >= 1 && opcion <= 4) { if (opcion == 1) { String textoMenuDia = "MENU DEL DIA DE HOY\n"; //VARIABLE QE CUENTA LOS REGISTROS int cont = 0; //OBTENEMOS EL DIA DE HOY LocalDate today = LocalDate.now(); DayOfWeek dayOfWeek = today.getDayOfWeek(); //SACACAMOS DACA REGISTRO for (String[] registro : registrosMenu) { //COMENZAMOS A PARTIR DEL 3 ER REGISTRO if (cont >= 2) { //OBTENEMOS EL DIA DE HOY EN NUMERO int diaSemanaNum = dayOfWeek.getValue(); //OBTENEMOS LA COLUMNA DEL PRECIO int columnaPrecio = diaSemanaNum * 2; //OBTENEMOS LA COLUMNA DE LA COMIDA int columnaComida = (diaSemanaNum * 2) + 1; //OBTENEMOS LA COMIDA String comida = registro[columnaPrecio]; //OBTENEMOS EL PRECIO String precio = registro[columnaComida]; //CONCATENAMOS EL TEXTO textoMenuDia = textoMenuDia + comida + ": $" + precio + "\n"; } cont++; } //ESCRIBIMOS EL MENSAJE driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys(textoMenuDia); pausa(500); driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys(Keys.ESCAPE); etapa[usuarioSel] = 1; } if (opcion == 2) { String textoMenuOtroDia = "De que dia quiere saber el menu\n" + "1.-Lunes\n2.-Martes\n3.-Miercoles\n4.-Jueves\n5.-Viernes\n6.-Sabado\n7.-Domingo\n"; //ESCRIBIMOS EL MENSAJE driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys(textoMenuOtroDia); pausa(500); driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys(Keys.ESCAPE); etapa[usuarioSel] = 3; } if (opcion == 3) { String textoMenuHorarios = "HORARIOS\n" + lunes + "\n" + martes + "\n" + miercoles + "\n" + jueves + "\n" + viernes + "\n" + sabado + "\n" + domingo + "\n"; //ESCRIBIMOS EL MENSAJE driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys(textoMenuHorarios); pausa(500); driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys(Keys.ESCAPE); etapa[usuarioSel] = 1; } if (opcion == 4) { String textoMenuAcerca = "ACERCA DE NOSOTROS\n" + telefono + "\n" + direccion + "\n" + domicilio + "\n" + pagina + "\n"; //ESCRIBIMOS EL MENSAJE driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys(textoMenuAcerca); pausa(500); driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys(Keys.ESCAPE); etapa[usuarioSel] = 1; } } //NO SE SELECCIONO LA OPCION CORRECTA else { //ESCRIBIMOS EL MENSAJE driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys("Opcion no valida\n"); pausa(500); driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys(Keys.ESCAPE); etapa[usuarioSel] = 1; } } else { //ESCRIBIMOS EL MENSAJE driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys("Opcion no valida\n"); pausa(500); driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys(Keys.ESCAPE); etapa[usuarioSel] = 1; } } public void etapa3() { //VARIABLE QUE RECIBE LAS OPCIONES COLOCADAS POR EL USUARIO DE WA int opcion; //QUITAMOS ESPACIOS EN BLANCO String mensajesRecibidosSinEspacios = mensajesRecibidos[usuarioSel].replace(" ", ""); //VALIDAMO SI REALMENTE SE ENVIO UN NUMERO if (isNumeric(mensajesRecibidosSinEspacios)) { opcion = Integer.parseInt(mensajesRecibidosSinEspacios); //SI LA OPCION ESTA ENTRE 1 Y 7 if (opcion >= 1 && opcion <= 7) { String textoMenuDia = "MENU DEL DIA " + deNumeroAdiaSemana(opcion) + "\n"; //SI ESCRIBIO LA OPCION 7 LA RRESCRIBIMOS COMO 0 OSEA QUE EL DOMINGO ES EL DIA 0 if (opcion == 7) { opcion = 0; } //VARIABLE QE CUENTA LOS REGISTROS int cont = 0; //SACACAMOS DACA REGISTRO for (String[] registro : registrosMenu) { //COMENZAMOS A PARTIR DEL 3 ER REGISTRO if (cont >= 2) { //OBTENEMOS LA COLUMNA DEL PRECIO int columnaPrecio = opcion * 2; //OBTENEMOS LA COLUMNA DE LA COMIDA int columnaComida = (opcion * 2) + 1; //OBTENEMOS LA COMIDA String comida = registro[columnaPrecio]; //OBTENEMOS EL PRECIO String precio = registro[columnaComida]; //SI HAY TEXTO EN COMIDA ESCRIBIMOS LA LINEA if (comida.length() > 0) { //CONCATENAMOS EL TEXTO textoMenuDia = textoMenuDia + comida + ": $" + precio + "\n"; } } cont++; } //ESCRIBIMOS EL MENSAJE driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys(textoMenuDia); pausa(500); driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys(Keys.ESCAPE); etapa[usuarioSel] = 1; } else { //ESCRIBIMOS EL MENSAJE driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys("Opcion no valida\n"); pausa(500); driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys(Keys.ESCAPE); } } else { //ESCRIBIMOS EL MENSAJE driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys("Opcion no valida\n"); pausa(500); driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys(Keys.ESCAPE); } } public String deNumeroAdiaSemana(int dia) { if (dia == 1) { return "LUNES"; } if (dia == 2) { return "MARTES"; } if (dia == 3) { return "MIERCOLES"; } if (dia == 4) { return "JUEVES"; } if (dia == 5) { return "VIERNES"; } if (dia == 6) { return "SABADO"; } if (dia == 7) { return "DOMINGO"; } return ""; } //FUNCION QUE ESPERA UN TIEMPO public void pausa(long sleeptime) { try { Thread.sleep(sleeptime); } catch (InterruptedException ex) { } } //FUNCION QUE VALIDA SI LA CADENA DE TEXTO ES UN NUMERO public boolean isNumeric(String cadena) { try { Float.parseFloat(cadena); return true; } catch (NumberFormatException nfe) { return false; } } //Quitamos las lineas finales private String[] removeTrailingQuotes(String[] fields) { String QUOTE = "\""; String result[] = new String[fields.length]; for (int i = 0; i < result.length; i++) { result[i] = fields[i].replaceAll("^" + QUOTE, "").replaceAll(QUOTE + "$", ""); } return result; } private void leemosMenu() { try (CSVReader reader = new CSVReader(new FileReader(localPath + "\\menu.csv"))) { //VARIABLE QUE ALMACENARA UNA LINEA String[] linea; //NOS MOVEMOS LINEA POR LINEA PARA ALMACENAR TODOS OS REGISTROS EN EL ARREGLO while ((linea = reader.readNext()) != null) { registrosMenu.add(linea); } //etapa2(); } catch (IOException ex) { Logger.getLogger(ChatBotRestaurante.class.getName()).log(Level.SEVERE, null, ex); } } //CREAMOS UN ARREGLO QUE ALAMACENARA LOS DATOS DEL RESTAURANTE private void leemosDatos() { //CREAMOS UN ARREGLO QUE ALAMACENARA LOS REGISTROS DEL EXCEL List<String[]> registrosDatos = new ArrayList<>(); try (CSVReader reader = new CSVReader(new FileReader(localPath + "\\datos.csv"))) { //VARIABLE QUE ALMACENARA UNA LINEA String[] linea; //NOS MOVEMOS LINEA POR LINEA PARA ALMACENAR TODOS OS REGISTROS EN EL ARREGLO while ((linea = reader.readNext()) != null) { if (linea[0].equals("Saludo")) { saludo = linea[1]; } if (linea[0].equals("Teléfono")) { telefono = linea[0] + ": " + linea[1]; } if (linea[0].equals("Dirección")) { direccion = linea[0] + ": " + linea[1]; } if (linea[0].equals("Entregas a domicilio")) { domicilio = linea[0] + ": " + linea[1]; } if (linea[0].equals("Pagina")) { pagina = linea[0] + ": " + linea[1]; } if (linea[0].equals("Lunes")) { lunes = linea[0] + " " + linea[1]; } if (linea[0].equals("Martes")) { martes = linea[0] + " " + linea[1]; } if (linea[0].equals("Miércoles")) { miercoles = linea[0] + " " + linea[1]; } if (linea[0].equals("Jueves")) { jueves = linea[0] + " " + linea[1]; } if (linea[0].equals("Viernes")) { viernes = linea[0] + " " + linea[1]; } if (linea[0].equals("Sábado")) { sabado = linea[0] + " " + linea[1]; } if (linea[0].equals("Domingo")) { domingo = linea[0] + " " + linea[1]; } } } catch (IOException ex) { Logger.getLogger(ChatBotRestaurante.class.getName()).log(Level.SEVERE, null, ex); } }
Código completo:
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ package com.mycompany.chatbotrestaurante; import com.google.common.collect.Streams; import java.io.BufferedReader; import java.io.File; import java.io.FileReader; import java.io.IOException; import java.text.SimpleDateFormat; import java.time.LocalDateTime; import java.time.temporal.ChronoUnit; import java.util.ArrayList; import java.util.Arrays; import java.util.List; import java.util.Locale; import java.util.concurrent.TimeUnit; import java.util.logging.Level; import java.util.logging.Logger; import javax.swing.JOptionPane; import org.openqa.selenium.By; import org.openqa.selenium.JavascriptExecutor; import org.openqa.selenium.Keys; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.WindowType; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import org.openqa.selenium.support.ui.Select; import org.openqa.selenium.support.ui.WebDriverWait; import com.opencsv.CSVReader; import com.opencsv.CSVWriter; import java.time.DayOfWeek; import java.time.LocalDate; import java.util.NoSuchElementException; import io.github.bonigarcia.wdm.WebDriverManager; /** * * @author eucm2 */ public class ChatBotRestaurante extends javax.swing.JFrame { //DECLARAMOS EL WEBDRIVER QUE CONTROLARA EL CHROME WebDriver driver; //XPATH DEL AREA DE ESCRITURA DE WA String inputTextEnviarWA = "//*[@id=\"main\"]/footer/div[1]/div/span[2]/div/div[2]/div[1]/div/div[1]/p"; ArrayList<String> tabs; //NUMERO DE CONTACTOS QUE ESTAREMOS MANEJANDO int cantTabs = 0; //DIRECCION ACTUAL DEL PROGRAMA String localPath = ""; //*********MULTIUSUARIO******************* //LISTA DE CONTACTOS QUE MANEJAREMOS String[] contactos = new String[100]; int[] etapa = new int[100]; //USUARIO SELECCIONADO O TAB SELECCIONADA int usuarioSel = 0; //MENSAJES RECIBIDOS DESDE WA String[] mensajesRecibidos = new String[100]; /* VARIABLES DE EXCEL */ String saludo; String telefono; String direccion; String domicilio; String pagina; String lunes; String martes; String miercoles; String jueves; String viernes; String sabado; String domingo; //CREAMOS UN ARREGLO QUE ALAMACENARA LOS DATOS DEL MENU List<String[]> registrosMenu = new ArrayList<>(); public ChatBotRestaurante() { //OBTENEMOS LA DIRECCION ACTUAL DEL PROGRAMA try { localPath = new File(".").getCanonicalPath(); } catch (IOException ex) { Logger.getLogger(ChatBotRestaurante.class.getName()).log(Level.SEVERE, null, ex); } initComponents(); leemosMenu(); leemosDatos(); } public void abrirNavegador() { try { /* String SO = System.getProperty("os.name"); //SI EL SISTEMA OPERATIVO ES WINDOWS if (SO.equals("Windows 10")) { //LEEMOS EL CHROMEDRIVER QUE ESTA EN LA MISMA DIRECCION DEL PROGRAMA System.setProperty("webdriver.chrome.driver", localPath + "\\chromedriver.exe"); } else { System.setProperty("webdriver.chrome.driver", localPath + "/chromedriver"); } */ WebDriverManager.chromedriver().setup(); //INICIALIZAMOS LS OPCIONES DE CHROME ChromeOptions optionsGoo = new ChromeOptions(); //PERMITIMOS LA PROPIEDAD NO-SANDBOX PARA EVITAR PROBLEMAS EN LINUX optionsGoo.addArguments("--no-sandbox"); //DESHABILITAMOS LAS NOTIFICACIONES optionsGoo.addArguments("--disable-notifications"); optionsGoo.addArguments("Zoom 70%"); //GUARDAMOS LA SESION EN LA CARPETA CHROMEWA optionsGoo.addArguments("--user-data-dir=" + localPath + "\\chromeWA"); //INSTANCIAMOS UN NUEVO CHROMEDRIVER driver = new ChromeDriver(optionsGoo); driver.manage().timeouts().implicitlyWait(5, TimeUnit.SECONDS); //ABRIMOS WHATSAPP driver.get("https://web.whatsapp.com"); } catch (NoSuchElementException e) { Object[] options = {"Continuar", "Detener"}; int res = JOptionPane.showOptionDialog(null, "Desea continuar o detener? \n error= " + e, "Warning", JOptionPane.DEFAULT_OPTION, JOptionPane.WARNING_MESSAGE, null, options, options[0]); if (res == 1) { driver.quit(); } } catch (Exception e) { Object[] options = {"Continuar", "Detener"}; int res = JOptionPane.showOptionDialog(null, "Version de chrome no compatible porfavor descargue la version de chrome que corresponde \n error= " + e, "Warning", JOptionPane.DEFAULT_OPTION, JOptionPane.WARNING_MESSAGE, null, options, options[0]); if (res == 1) { driver.quit(); } } } public void activarWA() { //HORA EN LA QUE INICIO EL USUARIO while (jCheckBox1.isSelected() == true) { //REVISAMOS QUE EXISTAN NUEVO MENSAJES EN EL CHAT DE WA revisaWA(); pausa(1000); } } public void revisaWA() { //VARIABLE QUE ALMACENA LA TAB DE CHROME QUE NOS POSICIONAREMOS int tabSeleccionada = 0; //SI HAY UN MENSAJE EN WA if (driver.findElements(By.className("aumms1qt")).size() > 0) { //BUSCAMOS EL NOMBRE DEL CONTACTO String nombreContacto = driver.findElement(By.className("aumms1qt")).findElement(By.xpath("./../../../../..")).findElement(By.className("zoWT4")).getText(); //OBTENEMOS LA CANTIDAD MENSAJES ENVIADOS int cantNuevosMensajes = Integer.parseInt(driver.findElement(By.className("aumms1qt")).getText()); //DAMOS CLICK EN EL CONTACTO PARA ABRIR EL CHAT driver.findElement(By.className("aumms1qt")).findElement(By.xpath("./../../../../..")).findElement(By.className("zoWT4")).click(); //OBTENEMOS TODOS LOS MENSAJES List<WebElement> webElementsMsgWA = driver.findElements(By.className("_22Msk")); //OBTENEMOS TODAS LAS TABS tabs = new ArrayList<String>(driver.getWindowHandles()); boolean contactoEncontrado = false; //VALIDAMOS QUE EL NOMBRE DE CONTACTO NO ESTE EN LA LISTA DE CONTACTOS DE LO CONTRARIO NOS POSICIONAMOS EN EL ID DEL CONTACTO for (int tabSel = 1; tabSel <= cantTabs; tabSel++) { //SI EL CONTACTO YA EXISTE EN LA LISTA if (nombreContacto.equals(contactos[tabSel])) { //ACTIVAMOS LA BANDERA Y DECMOS QUE SI ENCONTRAMOS EL CONTACTO contactoEncontrado = true; usuarioSel = tabSel; //ROMPEMOS EL CICLO break; } } //SI NO SE ENCONTRO EL CONTACTO LO CREAMOS if (contactoEncontrado == false) { //AUMENTAMOS EL NUMERO DE CONTACTOS cantTabs++; //ASIGNAMOS EL NOMBRE DEL CONTACTO A LA LISTA DE CONTACTOS contactos[cantTabs] = nombreContacto; //OBTENEMOS EL ID DEL USUARIO SELECCIONADO usuarioSel = cantTabs; //ASIGANOS LA ETAPA DEL USUARIO QUE ES LA 1 (ENVIAR TEXTOS D ELOS SORTEOS) etapa[usuarioSel] = 1; } //VARIABLE QUE ALMACENARA LOS ULTIMOS MENSAJE ENVIADOS mensajesRecibidos[usuarioSel] = ""; //CICLO QUE UNIRA TODOS LOS ULTIMOS MENSAJES for (int i = cantNuevosMensajes; i >= 1; i--) { //OBTENEMOS CADA MENSAJE String ultimoMensaje = webElementsMsgWA.get(webElementsMsgWA.size() - i).findElement(By.className("i0jNr")).getText(); //UNIMOS LOS MENSAJES mensajesRecibidos[usuarioSel] = mensajesRecibidos[usuarioSel] + " " + ultimoMensaje; } try { if (etapa[usuarioSel] == 1) { //ETAPA 1 DONDE SE ENVIAN LOS MENSAJES DE LOS DIFERENTES SORTEOS etapa1(); return; } else if (etapa[usuarioSel] == 2) { //VALIDAMOS QUE SI SEA UN NUMERO DEL SORTEO Y PREGUNTAMOS SI QUIERE UN NUMERO AL AZAR Y QUIERE SELECCIONAR SU NUMERO //SI NO LLAMAMOS LA ETAPA 1 PARA QUE ENVIE POR WA LOS SORTEOS etapa2(); return; } if (etapa[usuarioSel] == 3) { //VALIDAMOS QUE SI SEA UN NUMERO DEL SORTEO Y PREGUNTAMOS SI QUIERE UN NUMERO AL AZAR Y QUIERE SELECCIONAR SU NUMERO //SI NO LLAMAMOS LA ETAPA 2 PARA QUE ENVIE POR WA LOS SORTEOS etapa3(); return; } } catch (Exception e) { java.util.logging.Logger.getLogger(ChatBotRestaurante.class.getName()).log(java.util.logging.Level.SEVERE, null, e); } } else { } } //Etapa 1 Donde se envian los mensajes de los diferentes sorteos public void etapa1() { //ABRIMOS EL TAB DEL CONTACTO String listaOpciones = saludo + "\n1.-Menu del dia de hoy\n2.-Menu de otro dia\n3.-Horarios\n4.-Acerca de nosotros\n"; //ESCRIBIMOS EL MENSAJE driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys(listaOpciones); pausa(500); //CERRAMOS CHAT DE WA driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys(Keys.ESCAPE); //PASAMOS A LA SIGUIENTE ETAPA etapa[usuarioSel] = 2; } public void etapa2() { //VARIABLE QUE RECIBE LAS OPCIONES COLOCADAS POR EL USUARIO DE WA int opcion; //QUITAMOS ESPACIOS EN BLANCO String mensajesRecibidosSinEspacios = mensajesRecibidos[usuarioSel].replace(" ", ""); //String mensajesRecibidosSinEspacios = "2"; //VALIDAMO SI REALMENTE SE ENVIO UN NUMERO if (isNumeric(mensajesRecibidosSinEspacios)) { opcion = Integer.parseInt(mensajesRecibidosSinEspacios); //SI LA OPCION ESTA ENTRE 1 Y 4 if (opcion >= 1 && opcion <= 4) { if (opcion == 1) { String textoMenuDia = "MENU DEL DIA DE HOY\n"; //VARIABLE QE CUENTA LOS REGISTROS int cont = 0; //OBTENEMOS EL DIA DE HOY LocalDate today = LocalDate.now(); DayOfWeek dayOfWeek = today.getDayOfWeek(); //SACACAMOS DACA REGISTRO for (String[] registro : registrosMenu) { //COMENZAMOS A PARTIR DEL 3 ER REGISTRO if (cont >= 2) { //OBTENEMOS EL DIA DE HOY EN NUMERO int diaSemanaNum = dayOfWeek.getValue(); //OBTENEMOS LA COLUMNA DEL PRECIO int columnaPrecio = diaSemanaNum * 2; //OBTENEMOS LA COLUMNA DE LA COMIDA int columnaComida = (diaSemanaNum * 2) + 1; //OBTENEMOS LA COMIDA String comida = registro[columnaPrecio]; //OBTENEMOS EL PRECIO String precio = registro[columnaComida]; //CONCATENAMOS EL TEXTO textoMenuDia = textoMenuDia + comida + ": $" + precio + "\n"; } cont++; } //ESCRIBIMOS EL MENSAJE driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys(textoMenuDia); pausa(500); driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys(Keys.ESCAPE); etapa[usuarioSel] = 1; } if (opcion == 2) { String textoMenuOtroDia = "De que dia quiere saber el menu\n" + "1.-Lunes\n2.-Martes\n3.-Miercoles\n4.-Jueves\n5.-Viernes\n6.-Sabado\n7.-Domingo\n"; //ESCRIBIMOS EL MENSAJE driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys(textoMenuOtroDia); pausa(500); driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys(Keys.ESCAPE); etapa[usuarioSel] = 3; } if (opcion == 3) { String textoMenuHorarios = "HORARIOS\n" + lunes + "\n" + martes + "\n" + miercoles + "\n" + jueves + "\n" + viernes + "\n" + sabado + "\n" + domingo + "\n"; //ESCRIBIMOS EL MENSAJE driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys(textoMenuHorarios); pausa(500); driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys(Keys.ESCAPE); etapa[usuarioSel] = 1; } if (opcion == 4) { String textoMenuAcerca = "ACERCA DE NOSOTROS\n" + telefono + "\n" + direccion + "\n" + domicilio + "\n" + pagina + "\n"; //ESCRIBIMOS EL MENSAJE driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys(textoMenuAcerca); pausa(500); driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys(Keys.ESCAPE); etapa[usuarioSel] = 1; } } //NO SE SELECCIONO LA OPCION CORRECTA else { //ESCRIBIMOS EL MENSAJE driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys("Opcion no valida\n"); pausa(500); driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys(Keys.ESCAPE); etapa[usuarioSel] = 1; } } else { //ESCRIBIMOS EL MENSAJE driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys("Opcion no valida\n"); pausa(500); driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys(Keys.ESCAPE); etapa[usuarioSel] = 1; } } public void etapa3() { //VARIABLE QUE RECIBE LAS OPCIONES COLOCADAS POR EL USUARIO DE WA int opcion; //QUITAMOS ESPACIOS EN BLANCO String mensajesRecibidosSinEspacios = mensajesRecibidos[usuarioSel].replace(" ", ""); //VALIDAMO SI REALMENTE SE ENVIO UN NUMERO if (isNumeric(mensajesRecibidosSinEspacios)) { opcion = Integer.parseInt(mensajesRecibidosSinEspacios); //SI LA OPCION ESTA ENTRE 1 Y 7 if (opcion >= 1 && opcion <= 7) { String textoMenuDia = "MENU DEL DIA " + deNumeroAdiaSemana(opcion) + "\n"; //SI ESCRIBIO LA OPCION 7 LA RRESCRIBIMOS COMO 0 OSEA QUE EL DOMINGO ES EL DIA 0 if (opcion == 7) { opcion = 0; } //VARIABLE QE CUENTA LOS REGISTROS int cont = 0; //SACACAMOS DACA REGISTRO for (String[] registro : registrosMenu) { //COMENZAMOS A PARTIR DEL 3 ER REGISTRO if (cont >= 2) { //OBTENEMOS LA COLUMNA DEL PRECIO int columnaPrecio = opcion * 2; //OBTENEMOS LA COLUMNA DE LA COMIDA int columnaComida = (opcion * 2) + 1; //OBTENEMOS LA COMIDA String comida = registro[columnaPrecio]; //OBTENEMOS EL PRECIO String precio = registro[columnaComida]; //SI HAY TEXTO EN COMIDA ESCRIBIMOS LA LINEA if (comida.length() > 0) { //CONCATENAMOS EL TEXTO textoMenuDia = textoMenuDia + comida + ": $" + precio + "\n"; } } cont++; } //ESCRIBIMOS EL MENSAJE driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys(textoMenuDia); pausa(500); driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys(Keys.ESCAPE); etapa[usuarioSel] = 1; } else { //ESCRIBIMOS EL MENSAJE driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys("Opcion no valida\n"); pausa(500); driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys(Keys.ESCAPE); } } else { //ESCRIBIMOS EL MENSAJE driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys("Opcion no valida\n"); pausa(500); driver.findElement(By.xpath(inputTextEnviarWA)).sendKeys(Keys.ESCAPE); } } public String deNumeroAdiaSemana(int dia) { if (dia == 1) { return "LUNES"; } if (dia == 2) { return "MARTES"; } if (dia == 3) { return "MIERCOLES"; } if (dia == 4) { return "JUEVES"; } if (dia == 5) { return "VIERNES"; } if (dia == 6) { return "SABADO"; } if (dia == 7) { return "DOMINGO"; } return ""; } //FUNCION QUE ESPERA UN TIEMPO public void pausa(long sleeptime) { try { Thread.sleep(sleeptime); } catch (InterruptedException ex) { } } //FUNCION QUE VALIDA SI LA CADENA DE TEXTO ES UN NUMERO public boolean isNumeric(String cadena) { try { Float.parseFloat(cadena); return true; } catch (NumberFormatException nfe) { return false; } } //Quitamos las lineas finales private String[] removeTrailingQuotes(String[] fields) { String QUOTE = "\""; String result[] = new String[fields.length]; for (int i = 0; i < result.length; i++) { result[i] = fields[i].replaceAll("^" + QUOTE, "").replaceAll(QUOTE + "$", ""); } return result; } private void leemosMenu() { try (CSVReader reader = new CSVReader(new FileReader(localPath + "\\menu.csv"))) { //VARIABLE QUE ALMACENARA UNA LINEA String[] linea; //NOS MOVEMOS LINEA POR LINEA PARA ALMACENAR TODOS OS REGISTROS EN EL ARREGLO while ((linea = reader.readNext()) != null) { registrosMenu.add(linea); } //etapa2(); } catch (IOException ex) { Logger.getLogger(ChatBotRestaurante.class.getName()).log(Level.SEVERE, null, ex); } } //CREAMOS UN ARREGLO QUE ALAMACENARA LOS DATOS DEL RESTAURANTE private void leemosDatos() { //CREAMOS UN ARREGLO QUE ALAMACENARA LOS REGISTROS DEL EXCEL List<String[]> registrosDatos = new ArrayList<>(); try (CSVReader reader = new CSVReader(new FileReader(localPath + "\\datos.csv"))) { //VARIABLE QUE ALMACENARA UNA LINEA String[] linea; //NOS MOVEMOS LINEA POR LINEA PARA ALMACENAR TODOS OS REGISTROS EN EL ARREGLO while ((linea = reader.readNext()) != null) { if (linea[0].equals("Saludo")) { saludo = linea[1]; } if (linea[0].equals("Teléfono")) { telefono = linea[0] + ": " + linea[1]; } if (linea[0].equals("Dirección")) { direccion = linea[0] + ": " + linea[1]; } if (linea[0].equals("Entregas a domicilio")) { domicilio = linea[0] + ": " + linea[1]; } if (linea[0].equals("Pagina")) { pagina = linea[0] + ": " + linea[1]; } if (linea[0].equals("Lunes")) { lunes = linea[0] + " " + linea[1]; } if (linea[0].equals("Martes")) { martes = linea[0] + " " + linea[1]; } if (linea[0].equals("Miércoles")) { miercoles = linea[0] + " " + linea[1]; } if (linea[0].equals("Jueves")) { jueves = linea[0] + " " + linea[1]; } if (linea[0].equals("Viernes")) { viernes = linea[0] + " " + linea[1]; } if (linea[0].equals("Sábado")) { sabado = linea[0] + " " + linea[1]; } if (linea[0].equals("Domingo")) { domingo = linea[0] + " " + linea[1]; } } } catch (IOException ex) { Logger.getLogger(ChatBotRestaurante.class.getName()).log(Level.SEVERE, null, ex); } } /** * This method is called from within the constructor to initialize the form. * WARNING: Do NOT modify this code. The content of this method is always * regenerated by the Form Editor. */ @SuppressWarnings("unchecked") // <editor-fold defaultstate="collapsed" desc="Generated Code"> private void initComponents() { btnAbrirWA = new javax.swing.JButton(); jCheckBox1 = new javax.swing.JCheckBox(); setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE); btnAbrirWA.setText("Abrir WA"); btnAbrirWA.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { btnAbrirWAActionPerformed(evt); } }); jCheckBox1.setText("Activar chatbot"); jCheckBox1.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jCheckBox1ActionPerformed(evt); } }); javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane()); getContentPane().setLayout(layout); layout.setHorizontalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addGap(22, 22, 22) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false) .addComponent(jCheckBox1, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE) .addComponent(btnAbrirWA, javax.swing.GroupLayout.PREFERRED_SIZE, 132, javax.swing.GroupLayout.PREFERRED_SIZE)) .addContainerGap(246, Short.MAX_VALUE)) ); layout.setVerticalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addGap(25, 25, 25) .addComponent(btnAbrirWA, javax.swing.GroupLayout.PREFERRED_SIZE, 43, javax.swing.GroupLayout.PREFERRED_SIZE) .addGap(18, 18, 18) .addComponent(jCheckBox1, javax.swing.GroupLayout.PREFERRED_SIZE, 35, javax.swing.GroupLayout.PREFERRED_SIZE) .addContainerGap(179, Short.MAX_VALUE)) ); pack(); }// </editor-fold> private void btnAbrirWAActionPerformed(java.awt.event.ActionEvent evt) { abrirNavegador(); } private void jCheckBox1ActionPerformed(java.awt.event.ActionEvent evt) { activarWA(); } /** * @param args the command line arguments */ public static void main(String args[]) { /* Set the Nimbus look and feel */ //<editor-fold defaultstate="collapsed" desc=" Look and feel setting code (optional) "> /* If Nimbus (introduced in Java SE 6) is not available, stay with the default look and feel. * For details see http://download.oracle.com/javase/tutorial/uiswing/lookandfeel/plaf.html */ try { for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) { if ("Nimbus".equals(info.getName())) { javax.swing.UIManager.setLookAndFeel(info.getClassName()); break; } } } catch (ClassNotFoundException ex) { java.util.logging.Logger.getLogger(ChatBotRestaurante.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } catch (InstantiationException ex) { java.util.logging.Logger.getLogger(ChatBotRestaurante.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } catch (IllegalAccessException ex) { java.util.logging.Logger.getLogger(ChatBotRestaurante.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } catch (javax.swing.UnsupportedLookAndFeelException ex) { java.util.logging.Logger.getLogger(ChatBotRestaurante.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } //</editor-fold> /* Create and display the form */ java.awt.EventQueue.invokeLater(new Runnable() { public void run() { new ChatBotRestaurante().setVisible(true); } }); } // Variables declaration - do not modify private javax.swing.JButton btnAbrirWA; private javax.swing.JCheckBox jCheckBox1; // End of variables declaration }
Ave que vuela, a la cazuela.